What is a data type in Java?
In this article we are going to explain primitive data types in Java, which are the most basic data types in Java.
Table of Contents
1. What types do we have?
There are eight primitive data types in Java available:
- byte
- int
- short
- long
- float
- double
- boolean
- char
In sum we can say java primitive types contain simple values, which we know from real life.
But first we want to show you how the data types are divided shown in a graph:
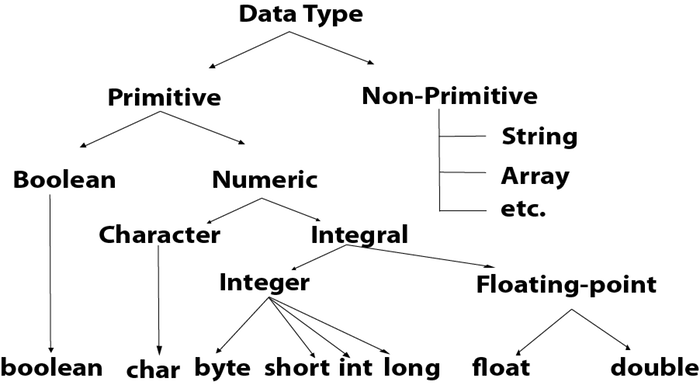
This graph (or mind map) shows us that Data Types are divided in 2 main sections, Primitive and Non-primitive.
Non-primitive data types(String array etc.) are called reference types in Java and they refer to an object. They are created by the programmer and are not defined by Java like Primitives are.
Primitive data types are divided to Boolean, which can only give 2 values True and False, and Numeric. When you hear "Numeric Data Type" you automatically think that it can give values that are only numbers, well that is not completely true. Numeric data types are divided on Character and Integral. Character type can only give us one character as an output (for example: a or b, or c) but it can give us letters, while Integral data type is divided in 2 sections, Integer and Floating-point. Integer contains byte, short, int and long data types, and Floating-point contains float and double. Look at the table below and you will see what are the values for each data type.
2. Java numbers
The most known data types for computers are of course numbers. But how are they represented?
We can answer that with a table that shows what are the values of each data type:
Reserved keyword | Data Type | Size | Range of Values |
---|---|---|---|
byte | Byte Length Integer | 1 byte | -28 to 27-1 |
short | Short Integer | 2 bytes | -216 to 216-1 |
int | Integer | 4 bytes | -232 to 231-1 |
long | Long Integer | 8 bytes | -264 to 263-1 |
float | Single Precision | 4 bytes | -231 to 231-1 |
double | Real number with double | 8 bytes | -264 to 262-1 |
char | Character (16 bit unicode) | 2 bytes | 0 to 216-1 |
boolean | Has value true of false | A boolean value | true or false |
2.1. The data type: Java byte
Java byte is a type of data that contains only numbers without fractional a component (byte values for example: 2, 12, 48, 13, -58). Since the name byte, also Java uses only 8 bit of the memory to store the information of this data type. Byte can only hold the values from -27 to 27–1. So the Java byte max values are -128 and 127. The value 0 is defined a positive value and therefore we have only 127 as a maximum positive value.
The default value of a initialized byte is 0
We declare byte like this:
public class ExampleByte {
public static void main(String[] args) {
byte b = 100;
System.out.println(b);
}
}
The result of this example would be:
100
Here is the screenshot from the Eclipse:
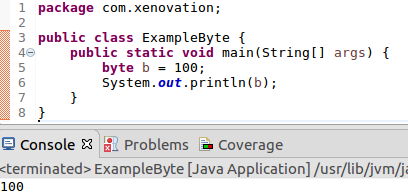
2.2. The data type: Java int also known as integer
Very similar to the data type byte, Java int is a type of data that contains only numbers without fractional component. Java stores it using 32 bits of memory. In other words, it can represent values from -231 to 231-1. So the Java integer max values are -2,147,483,648 and 2,147,483,647.
It's default value is 0.
We declare the java int data type like this:
public class ExampleInt {
public static void main(String[] args) {
int x = 424242;
System.out.println(x);
}
}
The result of this example would be:
424242
Here is the screenshot from the Eclipse:
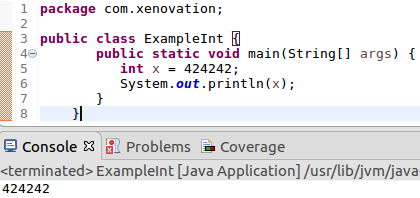
2.3. The data type: Java short
Short is also related to int, and by memory size it is placed in between int and byte. It is stored in 16 bits of memory and therefore stores values from -32,768 (-215 ) to 32,767 (215-1). So the Java short max values are -32,768 and 32,767.
Same as byte and int its default value is 0
We declare it like this:
public class ExampleShort {
public static void main(String[] args) {
short s = (short) 202_020;
System.out.println(s);
}
}
The result of this example would be:
5412
Here is the screenshot from the Eclipse:
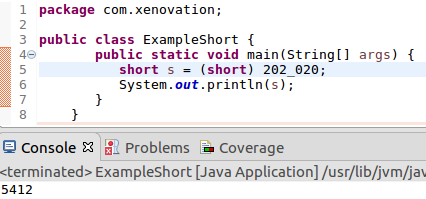
2.4. The data type: Java long
Long is last primitive type related to int, it is stored in 64 bits of memory, which means it can store more values than integer, stores values from (-263) to (263-1). So the Java long max values are -9,223,372,036,854,775,808 and 9,223,372,036,854,775,807.
Although long is related to integer its default value is 0 but 0L
We declare it like this:
public class ExampleLong {
public static void main(String[] args) {
long l = 1_234_567_890;
System.out.println(l);
}
}
The result of this example would be:
1234567890
Here is the screenshot from the Eclipse:
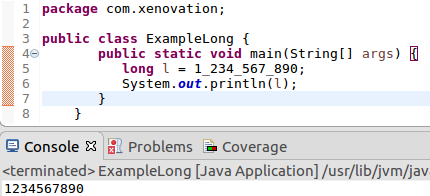
2.5. The data type: Java float
This is a single-precision decimal number. Which means if we get past six decimal points, this number becomes less precise and more of an estimate. It is stored in 32 bits of memory, which means it can contain values same as integer.
Floats default value is 0.0f
We declare it like this:
public class ExampleFloat {
public static void main(String[] args) {
float f = 3.145f;
System.out.println(f);
}
}
The result of this example would be:
3.145
Here is the screenshot from the Eclipse:
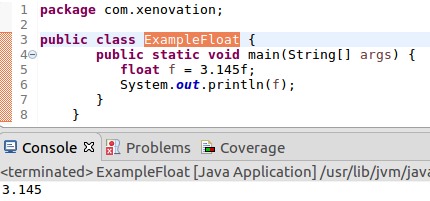
2.6. The data type: Java double
Double is expanded float, and its name comes from the fact that it’s a double-precision decimal number. It is stored in 64 bits of memory, same as long.
The default value of double is 0.0d.
We declare it like this:
public class ExampleDouble {
public static void main(String[] args) {
double d = 3.13457599923384753929348D;
System.out.println(d);
}
}
The result of this example would be:
3.1345759992338476
Here is the screenshot from the Eclipse:
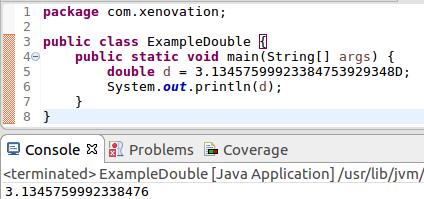
2.7. The data type: Java boolean
The simplest primitive data type is boolean. It can contain only two values: true or false. It stores its values in a single bit. It is useful for simple true/false statements.
Its default value is “false”.
We declare it like this:
public class ExampleBoolean {
public static void main(String[] args) {
boolean b = true;
System.out.println(b);
}
}
The result of this example would be:
true
Here is the screenshot from the Eclipse:
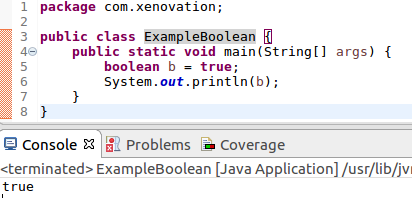
3. Text representing Java data types
There are data types that are representing characters / text, and they are:
3.1. The data type: Java char
Also called a character, char is 16-bit integer representing a Unicode-encoded character. Its range is from 0 to 65.535. In char we can only type single character, that is why it is primitive type and not like string, which is class, where we can type words.
Default value for char is "\u0000"
It is declared like this:
public class ExampleChar {
public static void main(String[] args) {
char c = 'a';
System.out.println(c);
}
}
The result of this example would be:
a
Here is the screenshot from the Eclipse:
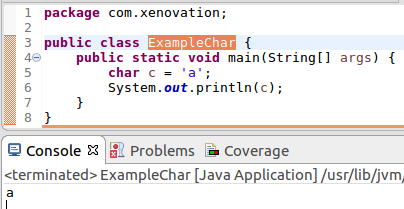
3.2. The data type: Java string
The String class represents character strings. All string literals in Java programs, such as "Hello" are implementer as instances of this class. Strings are constant, their values cannot be changed after they are created.
Default value for a Java string is null.
Here are some samples of how string can be used:
public class ExampleString {
public static void main(String[] args) {
System.out.println("Hello");
String str = "cde";
System.out.println("abc" + str);
}
}
The result of this example would be:
Hello
abccde
Here is the screenshot from the Eclipse:
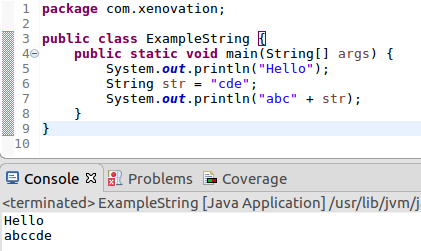
Great so far, but you have now more questions?