Rounding Number in Java
What is Rounding?
While we work with numbers in our program we come across the need to round the numbers. The rounding operation could be needed to round off the number before using it for the next operations or before saving it to the database or files.
In this article we will look into what is rounding, how does it work, and how do we achieve rounding in the Java programming language.
Rounding in general terms stands for transforming a number, by keeping it close to the original number.
Let's understand how this works
Table of Contents
- Rounding an integer number manually
- Rounding a decimal number manually
- Rounding Method in Java
- How do you round to the nearest integer in Java?
- Does Java round up or down?
- Dividing two integers to double in Java?
- How do you find the remainder in Java?
- How to round numbers up and down in Java?
- How do you round to 2 decimal places in Java?
- Conclusion
1. Rounding an integer number manually
Let us assume you want to round the number 82 to it's nearest ten value. Like learned in school the result would be 80. But what is the rounding algorithm behind it?
We will walk through the rounding logic step by step,
- Let's say we want to round the number 82 to its nearest TEN.
- Since we are rounding number 82 to nearest TEN, so the digit to keep is digit in the tens place.
- This will work out to be 8 in our case.
- The digit to keep is 8 and the next digit to it is 2.
- The next digit is 2, which is less than 5. So we keep the digit 8 AS IS.
- and we set the digit 2 to the value 0 (rounding down)
- as result we get 80
The general steps to follow to round any integer are as below,
- Identify a number to be rounded.
- identify the number to keep.
- Identify the digit to the left of the digit we need to keep. let's call it next digit
- If the "next" digit is less than 5, keep the digit as is
(this is also called rounding down). - If the "next" digit is greater than or equal to 5 than we increase
the digit to keep by 1 (this is also called rounding up)
The number 82, rounded to its nearest ten is 80, due to rounding down operation:
Number | Nearest | Number to Keep | Next Digit | Test | Rounded Number | Rounding |
82 | TEN | 8 | 2 | 2<5 | 80 | (Down) |
87 | TEN | 8 | 7 | 7>=5 | 90 | (Up) |
110 | HUNDRED | 1 | 1 | 1<5 | 100 | (Down) |
279 | HUNDRED | 2 | 7 | 7>=5 | 300 | (Up) |
See also here the table as illustrations:
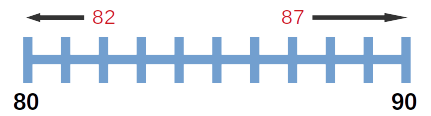

2. Rounding a decimal number manually
Let us assume you want to round the number 11.8231 to it's nearest tenth value. Like learned in school the result would be 11.80. But what is the rounding algorithm behind it?
In the case of decimal number,
- Rounding to nearest tenth means one digit after the decimal.
- Rounding to nearest hundredth mean two digits after the decimal and so on.
Now we will walk through the rounding logic step by step,
- Let's say we want to round the number 11.8231 to its nearest TENTH.
- Since we are rounding number 11.8231 to nearest TENTH,
so the number to keep is one digit after the decimal. - This will work out to be 8 in our case.
- The digit to keep is 8 and the next digit left to it is 2.
- The next digit is 2, which is less than 5. So we keep the digit 8 AS IS.
The general steps to follow to round any decimal number are as below,
- Identify a number to be rounded.
- Identify the digit to keep.
- Identify the digit left to the keep digit. let's call it the next digit.
- If the next digit is less than 5, keep the digit as is (this is also called rounding down).
- If the next digit is greater than or equal to 5 than we increase the digit to keep by 1 (this is also called rounding up)
The number 11.8231, rounded to its nearest tenth is 11.80, due to rounding down operation
Number | Nearest | Number to Keep | Next Digit | Test | Rounded Number | Rounding |
11.8231 | TENTH | 8 | 2 | 2<5 | 11.80 | (Down) |
12.87 | TENTH | 8 | 7 | 7>=5 | 12.90 | (Up) |
12.324 | HUNDREDTH | 2 | 4 | 4<5 | 12.320 | (Down) |
13.279 | HUNDREDTH | 7 | 9 | 9>=5 | 13.280 | (Up) |
See also here the table as illustrations:
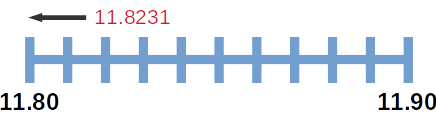
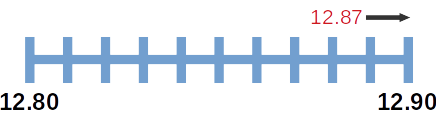
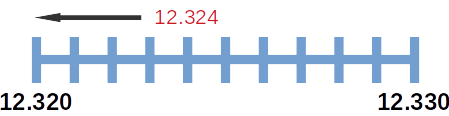
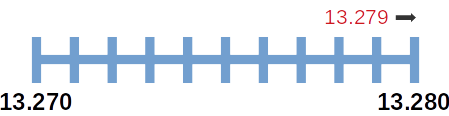
After refreshing our basic understanding let’s get into details of how Java deals with rounding.
3. Rounding Method in Java
The Java language provides a static round method from the java.lang.Math class.
The round method is an overloaded method with the following signatures.
- static long round(double d)
- static int round(float f)
Both get executed in code with Math.round()
4. How do you round to the nearest integer in Java?
The rounding method returns the nearest number based on the argument.
package com.xenovation.demo; import java.lang.Math; public class FloatNumberDemo { public static void main(String args[]) { float example1 = 1266.914f; float example1Rounded = Math.round(example1); System.out.printf("Example 1# %s ---> %s %n", example1, example1Rounded); float example2 = 1266.123f; float example2Rounded = Math.round(example2); System.out.printf("Example 2# %s ---> %s %n", example2, example2Rounded); float example3 = -3121.124f; float example3Rounded = Math.round(example3); System.out.printf("Example 3# %s ---> %s %n", example3, example3Rounded); float example4 = -3121.524f; float example4Rounded = Math.round(example4); System.out.printf("Example 4# %s ---> %s %n", example4, example4Rounded); } }
Output
Example 1# 1266.914 ---> 1267.0 Example 2# 1266.123 ---> 1266.0 Example 3# -3121.124 ---> -3121.0 Example 4# -3121.524 ---> -3122.0
5. Does Java round up or down?
Every one of us knows how the division of number works, so let's look at a set of examples,
- If we divide the number 13 by the number 4, we know the result would be 3.25.
- If we divide the number 15 by the number 4, we know the result would be 3.75.
When we divide two integers in Java, the output is always an integer.
If we run the code below the output shows the integer result. The result is 3 for both the division operation.
package com.xenovation.demo; public class NumberDivisionDemo { public static void main(String args[]) { int number1 = 13; int divisor = 4; int quotient1 = number1 / divisor; System.out.printf("Result of (%d/%d) is %d\n", number1, divisor, quotient1); int number2 = 15; int quotient2 = number2 / divisor; System.out.printf("Result of (%d/%d) is %d\n", number2, divisor, quotient2); } }
Output
Result of (13/4) is 3 Result of (15/4) is 3
If we apply the rounding concept the result should have been,
- (13/4) as 3.25 -- rounded to 3.
- (15/4) as 3.75 -- rounded to 4.
Which is not the output of the code above, since the Java picks the result in an integer.
Java internally does rounding to zero, remove anything to the right of the decimal when you divide two integers.
So Does Java division round down? The answer is Yes.
Java does a round down in case of division of two integer numbers.
6. Dividing two integers to double in Java?
As we saw in the previous section dividing two integers and getting the result in a integer variable, leads to a Round to zero behaviour. if you need a double result of the division type cast first the operands to a double type.
Let's check this out with an example.
package com.xenovation.demo; public class NumberDivisionDoubleResult { public static void main(String args[]) { int dividend = 10; int divisor = 3; double result = dividend / divisor; System.out.println("Dividing two integer and getting result in double."); System.out.printf("(%s/%s)=%s\n", dividend, divisor, result); System.out.println("Type casting dividend to double and getting result in double"); double result1 = ((double) dividend) / divisor; System.out.printf("(%s/%s)=%s", dividend, divisor, result1); } }
Dividing two integer and getting result in double. (10/3)=3.0 Typecasting dividend to double and getting result in double (10/3)=3.3333333333333335
7. How do you find the remainder in Java?
While we understand the division of two integer number in java, we get a round to zero behavior.
This does not mean we lost out the decimal portion.
If we wish to find the remainder of the division operation we use the % modulus operator.
package com.xenovation.demo; public class NumberRemainderDemo { public static void main(String args[]) { int divisor = 4; int number1 = 13; int number1Remainder = number1 % divisor; System.out.printf("Remainder of (%d/%d) is %d\n", number1, divisor, number1Remainder); int number2 = 15; int number2Remainder = number2 % divisor; System.out.printf("Remainder of (%d/%d) is %d\n", number2, divisor, number2Remainder); } }
Output
Remainder of (13/4) is 1 Remainder of (15/4) is 3
8. How to round numbers up and down in Java?
Java comes with two methods Math.ceil and Math.floor which round the number up and down respectively.
The code show usage of this two methods.
package com.xenovation.demo; import java.lang.Math; public class MathCeilFloorDemo { public static void main(String args[]) { double x = 32.333; double y = 98.893; double ceilX = Math.ceil(x); double ceilY = Math.ceil(y); double floorX = Math.floor(x); double floorY = Math.floor(y); System.out.printf("floor(%s) = %s\n", x, floorX); System.out.printf("floor(%s) = %s\n", y, floorY); System.out.printf("ceil(%s) = %s\n", x, ceilX); System.out.printf("ceil(%s) = %s\n", y, ceilY); } }
Output
floor( 32.333 ) = 32.0 floor( 98.893 ) = 98.0 ceil( 32.333 ) = 33.0 ceil( 98.893 ) = 99.0
8.1. What is Ceil and Floor in Java?
Let’s look at method definition and understand the rounding function in more detail.
At times, we want specific behavior for rounding up or rounding down.
Ceil and Floor are functions in Java which give you nearest integer down or up.
8.2. What does math Ceil do in Java?
static double ceil(double a)
The java math library provides a static ceil function which accepts a double.
The ceil method returns the smallest double value that is greater than or equal to equal to the argument and is equal to a mathematical integer.
8.3. What does math Floor do in Java?
static double floor(double a)
The java math library provides a static floor function which accepts a double.
The floor method returns the largest double value that is less than or equal to the argument and is equal to a mathematical integer.
9. How do you round to 2 decimal places in Java?
Many a times programmers are asked to round a decimal number to the last two signficant digits after the decimal.
Below code demonstrates how we can achieve the same.
package com.xenovation.demo; import java.math.BigDecimal; import java.math.RoundingMode; public class BigDecimalRoundDemo { /** * Performs a rounding operation on a double number, to the specified precision * * @param n * Holds the number to be formatted. * @param round2DecimalPlace * Holds the rounding precision. */ public static void roundAndPrint(double n, int round2DecimalPlace) { BigDecimal instance = new BigDecimal(Double.toString(n)); instance = instance.setScale(round2DecimalPlace, RoundingMode.HALF_UP); System.out.printf("%s rounded to %d decimal point = %s\n", n, round2DecimalPlace, instance.doubleValue()); } public static void main(String args[]) { roundAndPrint(10.1294, 2); roundAndPrint(10.1234, 2); roundAndPrint(10.1294, 3); roundAndPrint(10.1297, 3); } }
Output
10.1294 rounded to 2 decimal point = 10.13 10.1234 rounded to 2 decimal point = 10.12 10.1294 rounded to 3 decimal point = 10.129 10.1297 rounded to 3 decimal point = 10.13
10. Conclusion
Now you got a brief idea about the concept of rounding,
You now understand what is rounding down and rounding up, and the algorithm for rounding process
Also,you saw how rounding is done in Java and the readily available library functions.