Java final or Java static
In Java Final and Static keywords have a different roles, so let's explain them:
Java Final
Some more in depth explanation can be found also in What does java final mean?
Final is a keyword applicable to the class, variable and methods. As the word "Final" suggest it means that when we define something it is final, it cannot be changed. This also means that you must initialize a final variable. We must initialize a final variable, otherwise compiler will throw compile-time error. There are 3 ways to initialize a final variable:
- Initialize upon declaring, this is the most common approach, if it is not initialized upon declaring then we get, what is called, blank variable
- Blank variable can be initialized inside constructor. If you have more than one constructor in your class then it must be initialized in all of them, otherwise compile error will be thrown
- Blank variable can also be initialized inside static block
The method declared as final cannot be overridden by the subclass of that class in which final method is declared. And as for the classes, when they are declared as final, other classes cannot inherit that final class.
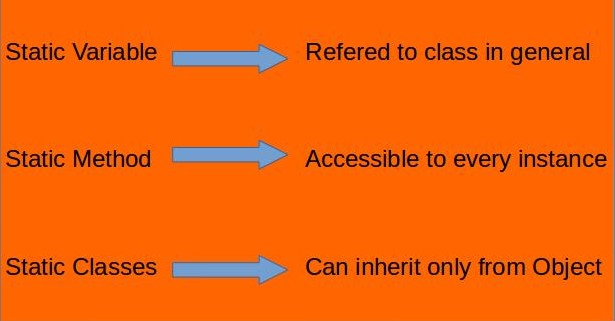
Java Static
The static keyword in Java is used for memory management. We can apply java static keyword with variables, methods, blocks and nested class.
- Static variables are global variables. All instance of the class share the same static variable, if static variable is declared as static, then a single copy of variable is created and shared to all objects at class level.
- When a method is declared with "static" keyword it is known as static method. The most common example of a static method is main() method. Any static member can be accessed before any objects of its class are created, and with out reference to any object.Methods declared as static have few restrictions:
- They can only directly call other static methods
- They can only directly access static data
- Java static block is used to initialize the static data member, it is executed before the main method at the time of class loading
- Nested classes are actually classes made inside of classes. They cannot access non-static data members and methods. Nested classes can access static data members of outer class including private.
When a class is declared as static, it becomes global for all other members of the class. You can access the static member of the class before its object is created. The best example of the static member is main() method, it is declared static so that it can be invoked before any object exist.
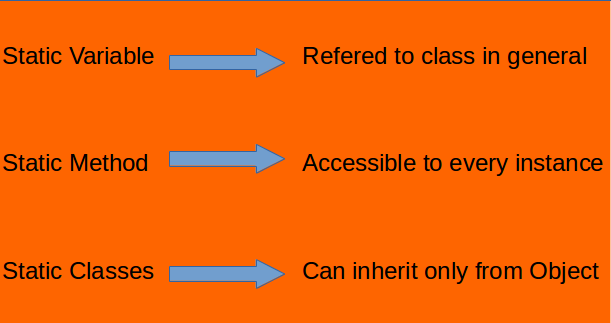
Key differences between Static and Final in Java
As we already explained them separately now just to summarize what are the main differences between the two of them:
- The static keyword is applicable to a nested static calss, variables, methods and blocks, while final keyword is applicable to class methods and variables.
- A static variable can be reinitialized where and whenever we want to, while final variable can never be reinitialized
- A static method can access the static member of the class and can only be invoked by other static methods, and final method can never be inherited by any class
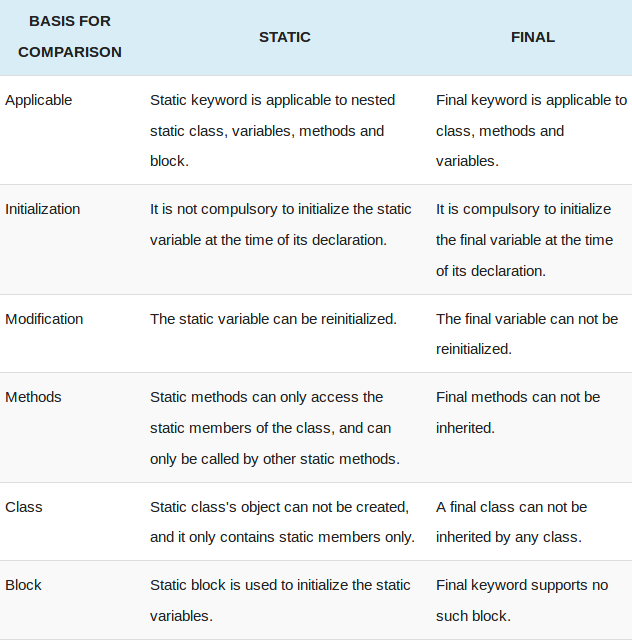
If you want to learn more about Java final click here